My previous Wordle helper has now been supplanted by a newer variant, which adds a number of nice new features. Here's a screenshot of the new UI:
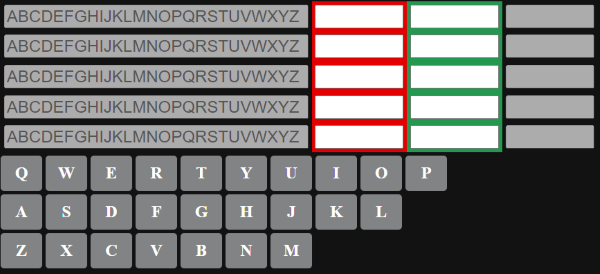
The top five rows represent the slots for each character of the five-letter word. Each row consists of four controls:
- The left-most column displays the possible remaining choices for that position.
- The column with a red background is where you specify letters that are in the word, but not in the given position.
- The column with a green background is where you specify letters that are in the word and are in the given position.
- The final column shows the subset of possibilities using only the letters known to be in the word.
As long as the body of the page has focus, you may simply type letters to remove them from play. Holding shift while typing a letter will add that letter to the pool of known letters. Holding the control key will remove the letter from the pool (if you make a mistake). Be careful with this, however; some browser shortcuts cannot reliably be trapped (ctrl + w being one of them).
As an alternative to typing, you can click the on-screen keyboard to remove letters, and Shift + click letters to add them. Here's a second screenshot showing the tool while in use:
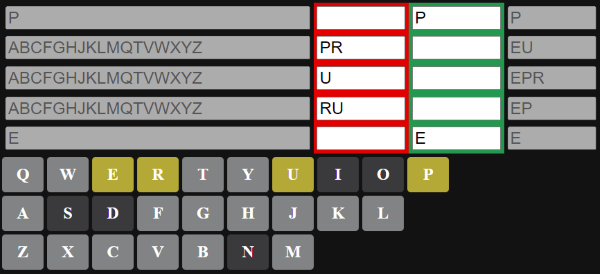